Hello friends welcome to another post,In this post we are going to make a telegarm and manual control homeautomation system with feedback,
we can control our homeappliances just by sending a text message from telegram from anywhere in the world and also we can switch ON or OFF Manually by regular switches as we alwaays do.
and the best part of this project is…..we can check the status of our homeappliances either it is ON or OFF just by sending text like light1state, light2state and so on.
Components Requried
1. ESP32
2. Highlink AC to DC Convertor(HLK-PMO1).
3. 5v Relay.
4. BC547 NPN Transistor.
5. IN4007 Diode.
6. Two pin Terminal Connector.
7. 1kohm & 10kohm Resistor.
8. Coustom Designed PCB.
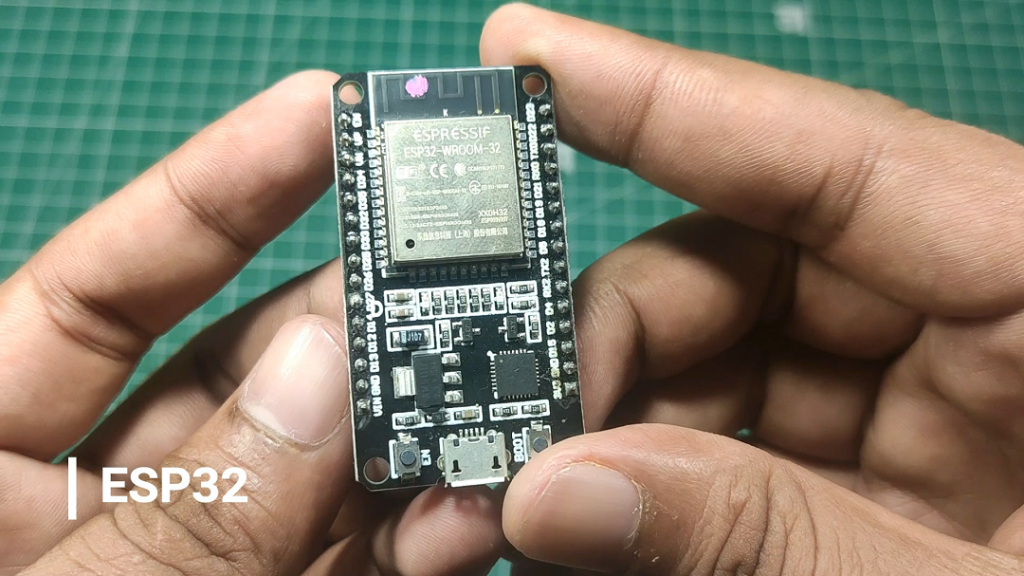
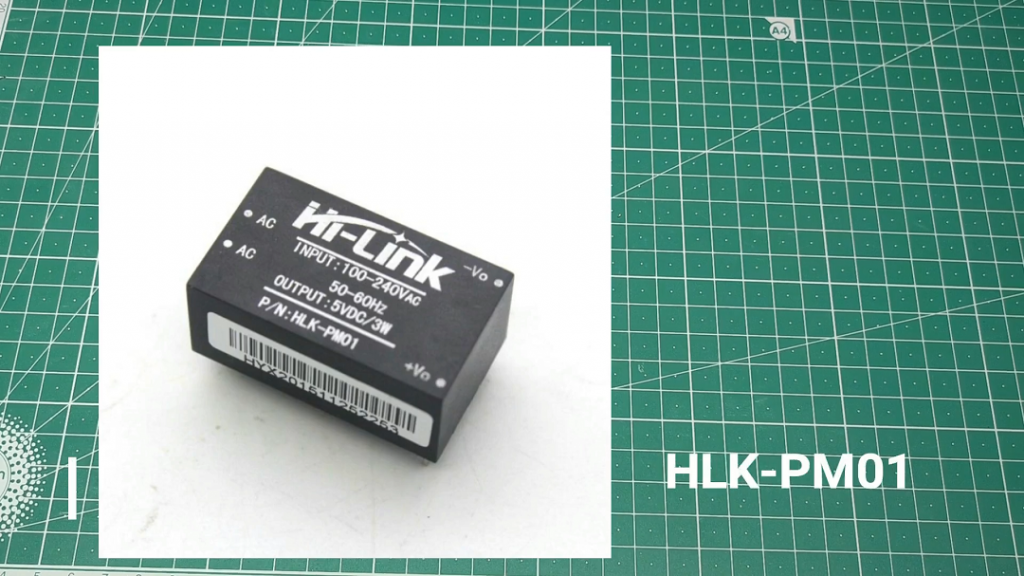
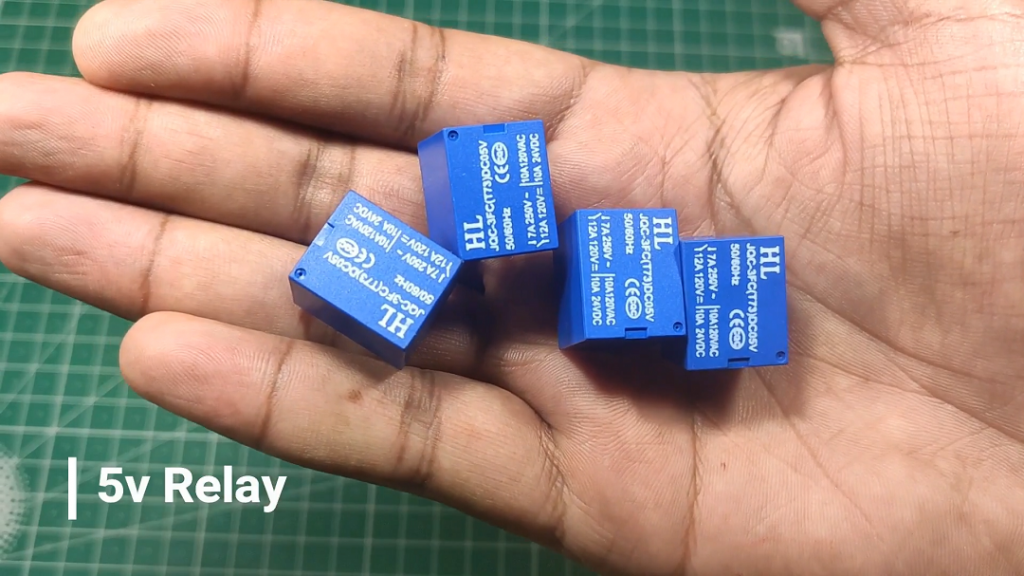
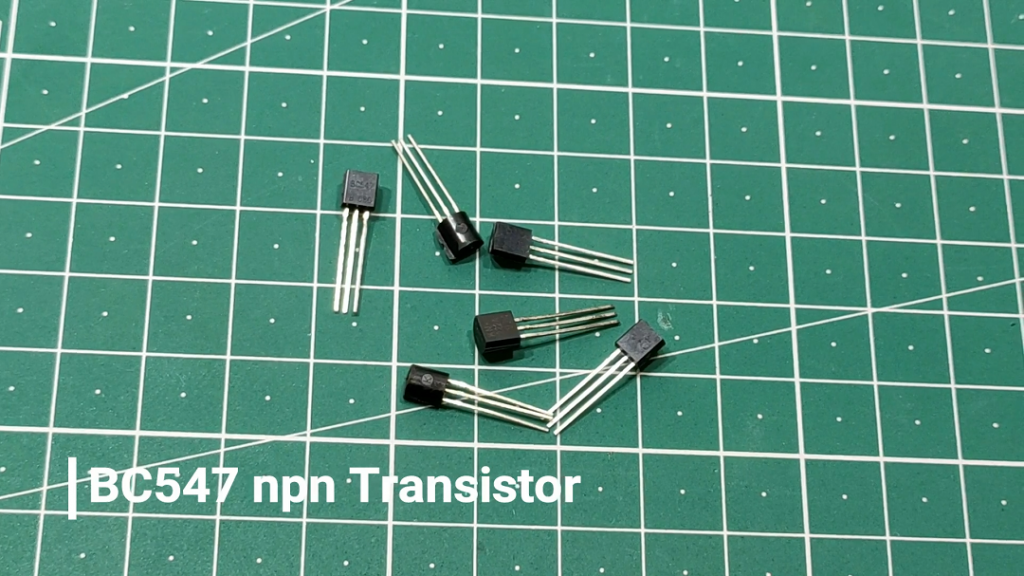
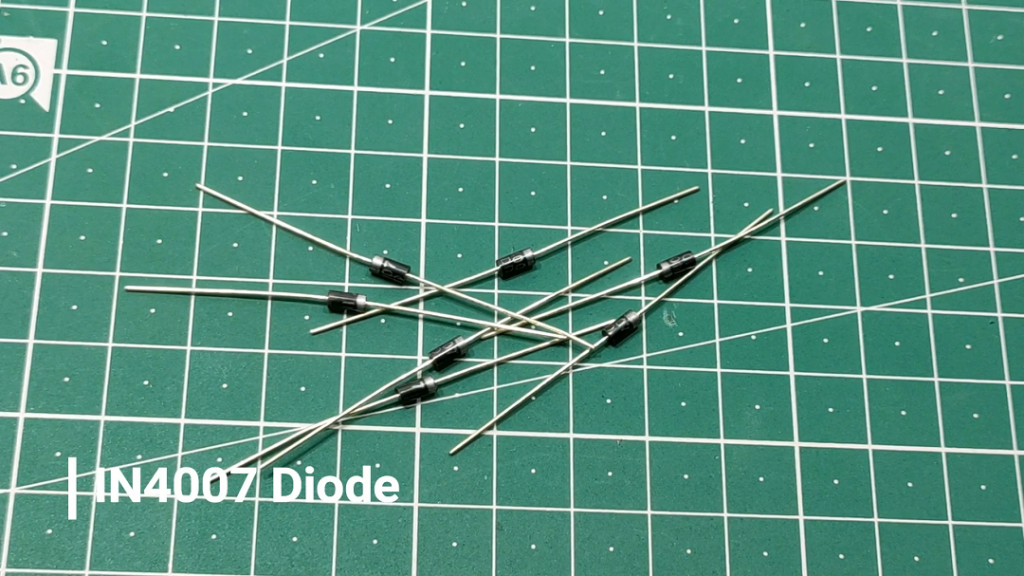
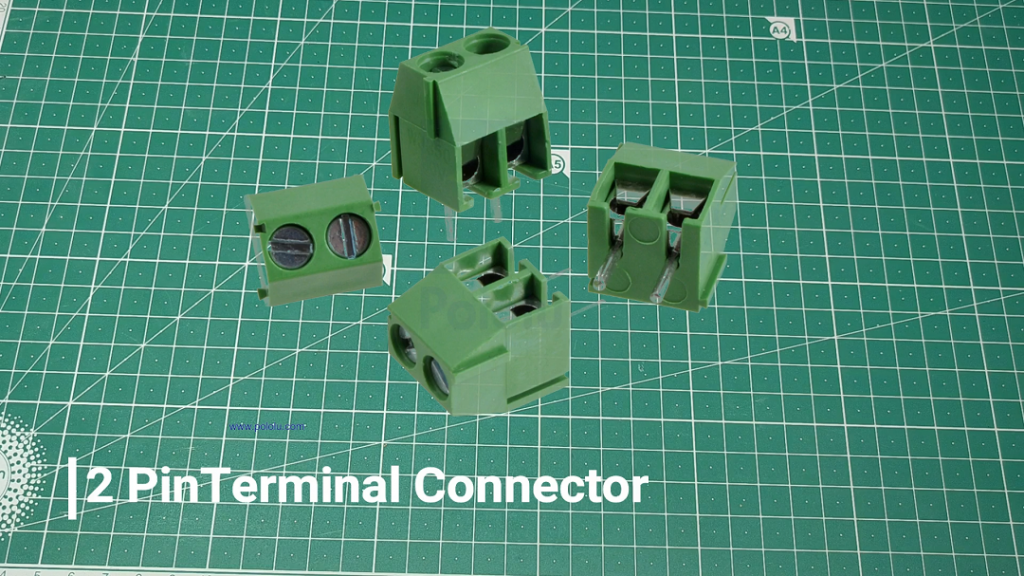
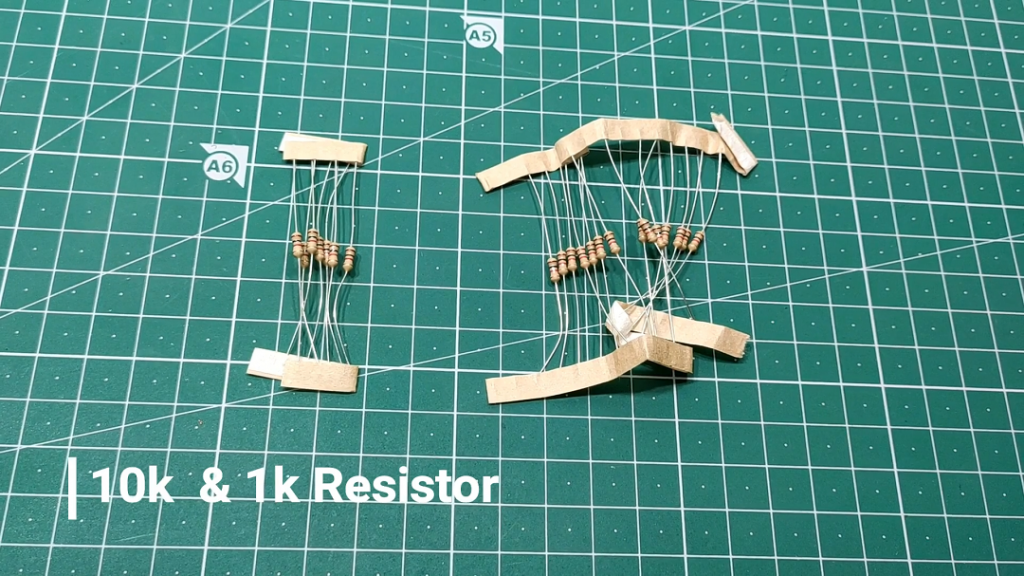
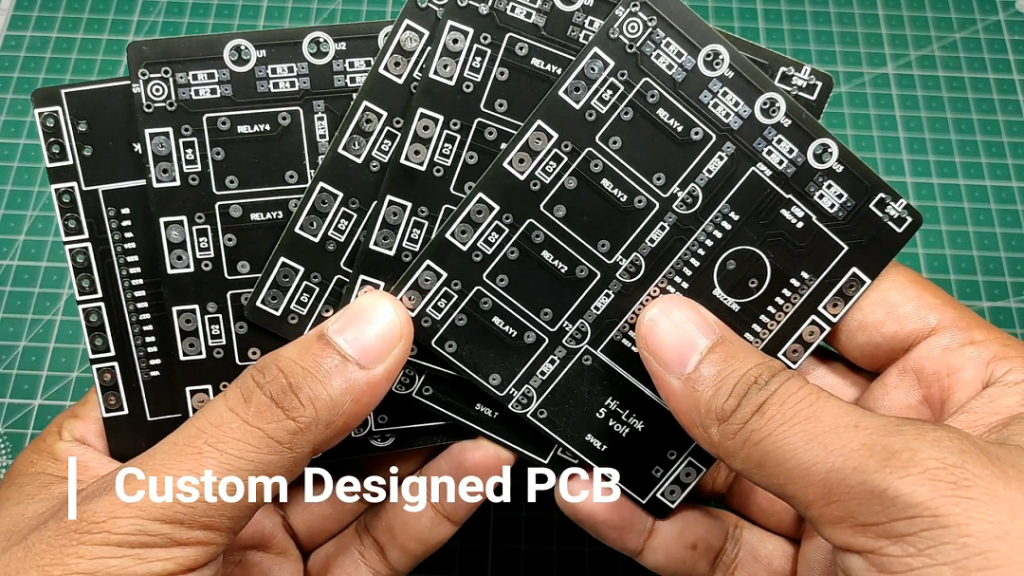
Connection of switches & bulbs
Connect all the switches and bulb as in the schematics shown below.
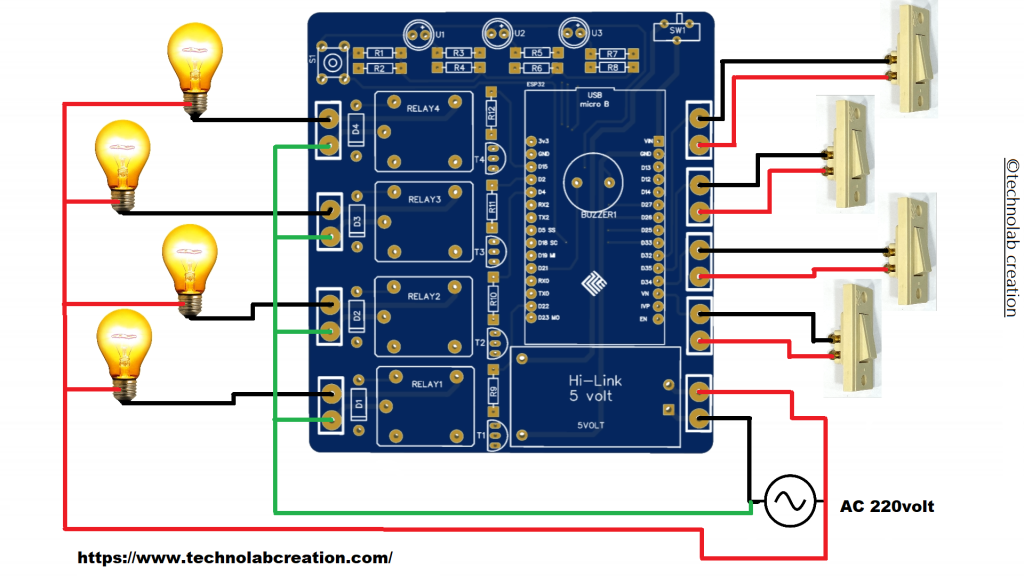
Code
#include <WiFi.h> #include <WiFiClientSecure.h> #include <UniversalTelegramBot.h> // Universal Telegram Bot Library written by Brian Lough: https://github.com/witnessmenow/Universal-Arduino-Telegram-Bot #include <ArduinoJson.h> // Replace with your network credentials const char* ssid = "xxxxxxxxxx"; const char* password = "xxxxxxxx"; // Initialize Telegram BOT #define BOTtoken "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" // your Bot Token (Get from Botfather) // Use @myidbot to find out the chat ID of an individual or a group // Also note that you need to click "start" on a bot before it can // message you #define CHAT_ID "xxxxxxx" WiFiClientSecure client; UniversalTelegramBot bot(BOTtoken, client); // Checks for new messages every 1 second. int botRequestDelay = 1000; unsigned long lastTimeBotRan; const int Relay1 = 15; const int Relay2 = 2; const int Relay3 = 4; const int Relay4 = 22; #define switch1 32 #define switch2 35 #define switch3 34 #define switch4 39 int switch_ON_Flag1_previous_I = 0; int switch_ON_Flag2_previous_I = 0; int switch_ON_Flag3_previous_I = 0; int switch_ON_Flag4_previous_I = 0; String Relay1State = "HIGH"; String Relay2State = "HIGH"; String Relay3State = "HIGH"; String Relay4State = "HIGH"; // Handle what happens when you receive new messages void handleNewMessages(int numNewMessages) { Serial.println("handleNewMessages"); Serial.println(String(numNewMessages)); for (int i=0; i<numNewMessages; i++) { // Chat id of the requester String chat_id = String(bot.messages[i].chat_id); if (chat_id != CHAT_ID){ bot.sendMessage(chat_id, "Unauthorized user", ""); continue; } // Print the received message String text = bot.messages[i].text; Serial.println(text); String from_name = bot.messages[i].from_name; text.toUpperCase(); delay(10); if (text == "START") { String welcome = "Welcome, " + from_name + ".\n"; welcome += "Use the following commands to control your outputs.\n\n"; welcome += "light1on to turn light1 ON \n"; welcome += "light1off to turn light1 OFF \n"; welcome += "light2on to turn light2 ON \n"; welcome += "light2off to turn light2 OFF \n"; welcome += "light3on to turn light3 ON \n"; welcome += "light3off to turn light3 OFF \n"; welcome += "light4on to turn light4 ON \n"; welcome += "light4off to turn light4 OFF \n"; // welcome += "/state to request current GPIO state \n"; bot.sendMessage(chat_id, welcome, ""); } if (text == "LIGHT1ON") { bot.sendMessage(chat_id, "Light1 state set to ON", ""); Relay1State = "ON"; digitalWrite(Relay1,LOW); } if (text == "LIGHT1OFF") { bot.sendMessage(chat_id, "Light1 state set to OFF", ""); Relay1State = "OFF"; digitalWrite(Relay1,HIGH); } if (text == "LIGHT2ON") { bot.sendMessage(chat_id, "Light2 state set to ON", ""); Relay2State = "ON"; digitalWrite(Relay2,LOW); } if (text == "LIGHT2OFF") { bot.sendMessage(chat_id, "Light2 state set to OFF", ""); Relay2State ="OFF"; digitalWrite(Relay2,HIGH); } if (text == "LIGHT3ON") { bot.sendMessage(chat_id, "Light3 state set to ON", ""); Relay3State = "ON"; digitalWrite(Relay3,LOW); } if (text == "LIGHT3OFF") { bot.sendMessage(chat_id, "Light3 state set to OFF", ""); Relay3State = "OFF"; digitalWrite(Relay3,HIGH); } if (text == "LIGHT4ON") { bot.sendMessage(chat_id, "Light4 state set to ON", ""); Relay4State = "ON"; digitalWrite(Relay4,LOW); } if (text == "LIGHT4OFF") { bot.sendMessage(chat_id, "Light4 state set to OFF", ""); Relay4State = "OFF"; digitalWrite(Relay4,HIGH); } ///////////////////////////////////////////////////////////////////////////////////// //////////////////////////////////// if (text == "LIGHT1STATE") { bot.sendMessage(chat_id, "Light1 is " + Relay1State + ""); } if (text == "LIGHT2STATE") { bot.sendMessage(chat_id, "Light2 is " + Relay2State + ""); } if (text == "LIGHT3STATE") { bot.sendMessage(chat_id, "Light3 is " + Relay3State + ""); } if (text == "LIGHT4STATE") { bot.sendMessage(chat_id, "Light4 is " + Relay4State + ""); } ///////////////////////////////////////////////////////////////////////////////////////////////////// } } void setup() { Serial.begin(115200); #ifdef ESP8266 client.setInsecure(); #endif pinMode(Relay1, OUTPUT); pinMode(Relay2, OUTPUT); pinMode(Relay3, OUTPUT); pinMode(Relay4, OUTPUT); pinMode(switch1, INPUT); pinMode(switch2, INPUT); pinMode(switch3, INPUT); pinMode(switch4, INPUT); digitalWrite(Relay1, LOW); digitalWrite(Relay2, LOW); digitalWrite(Relay3, LOW); digitalWrite(Relay4, LOW); // Connect to Wi-Fi WiFi.mode(WIFI_STA); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(1000); Serial.println("Connecting to WiFi.."); } // Print ESP32 Local IP Address Serial.println(WiFi.localIP()); } void loop() { ////////////////////////////////////////// if (digitalRead(switch1) == LOW) { if (switch_ON_Flag1_previous_I == 0 ) { digitalWrite(Relay1, LOW); Relay1State = "ON"; Serial.println("Relay1- ON"); switch_ON_Flag1_previous_I = 1; } } if (digitalRead(switch1) == HIGH ) { if (switch_ON_Flag1_previous_I == 1) { digitalWrite(Relay1, HIGH); Relay1State = "OFF"; Serial.println("Relay1 OFF"); switch_ON_Flag1_previous_I = 0; } } if (digitalRead(switch2) == LOW) { if (switch_ON_Flag2_previous_I == 0 ) { digitalWrite(Relay2, LOW); Relay2State = "ON"; Serial.println("Relay1- ON"); switch_ON_Flag2_previous_I = 1; } } if (digitalRead(switch2) == HIGH) { if (switch_ON_Flag2_previous_I == 1) { digitalWrite(Relay2, HIGH); Relay2State = "OFF"; Serial.println("Relay1 OFF"); switch_ON_Flag2_previous_I = 0; } } if (digitalRead(switch3) == LOW) { if (switch_ON_Flag3_previous_I == 0 ) { digitalWrite(Relay3, LOW); Relay3State = "ON"; Serial.println("Relay1- ON"); switch_ON_Flag3_previous_I = 1; } } if (digitalRead(switch3) == HIGH ) { if (switch_ON_Flag3_previous_I == 1) { digitalWrite(Relay3, HIGH); Relay3State = "OFF"; Serial.println("Relay1 OFF"); switch_ON_Flag3_previous_I = 0; } } if (digitalRead(switch4) == LOW) { if (switch_ON_Flag4_previous_I == 0 ) { digitalWrite(Relay4, LOW); Relay4State = "ON"; Serial.println("Relay1- ON"); switch_ON_Flag4_previous_I = 1; } } if (digitalRead(switch4) == HIGH ) { if (switch_ON_Flag4_previous_I == 1) { digitalWrite(Relay4, HIGH); Relay4State = "OFF"; Serial.println("Relay1 OFF"); switch_ON_Flag4_previous_I = 0; } } ///////////////////////////// if (millis() > lastTimeBotRan + botRequestDelay) { int numNewMessages = bot.getUpdates(bot.last_message_received + 1); while(numNewMessages) { Serial.println("got response"); handleNewMessages(numNewMessages); numNewMessages = bot.getUpdates(bot.last_message_received + 1); } lastTimeBotRan = millis(); } }
Copy this code and upload in your esp32 Board