Hello friends welcome to another post, In this post I will let you know how to make your own android app control homeautomation system using esp32. In this homeautomation system ,we are able to control our homeappliances with an android app as well as manually with regular switches as we always do, this two control homeautomation system is very easy and interesting project,
Components Required
1. ESP32
2. Highlink AC to DC Converter(HLK-PM01)
3. 5 volt Relay
4. BC 547 npn Transistor
5. IN 4007 Diode
6. 10k and 1k ohm Resistor
7. Two terminal connector
8. Custom Designed PCB.
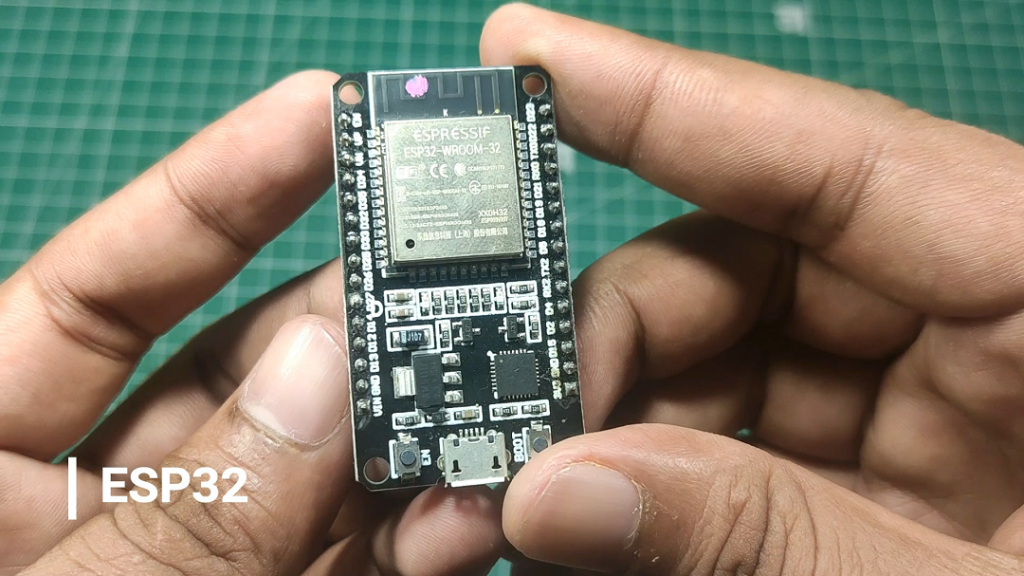
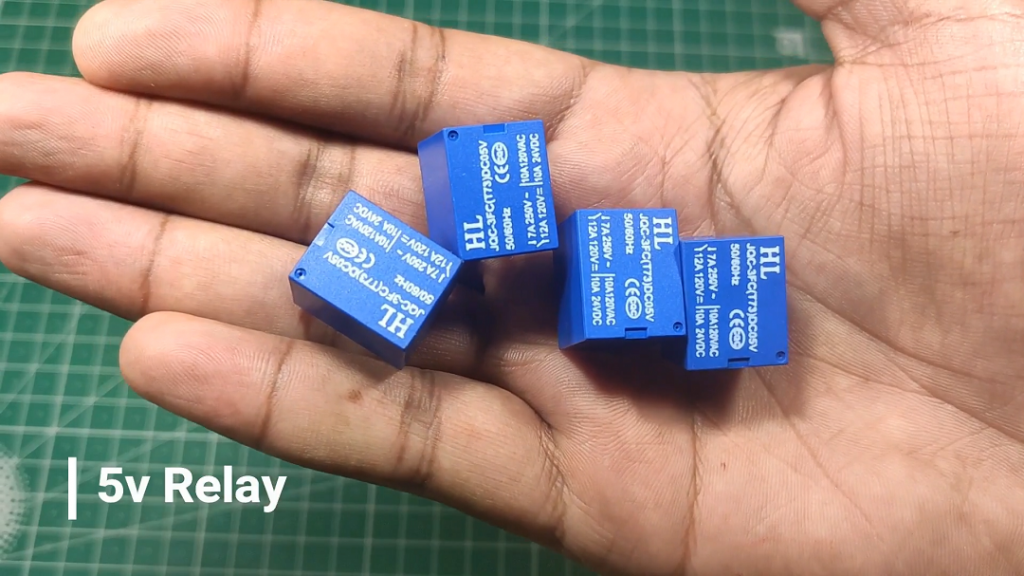
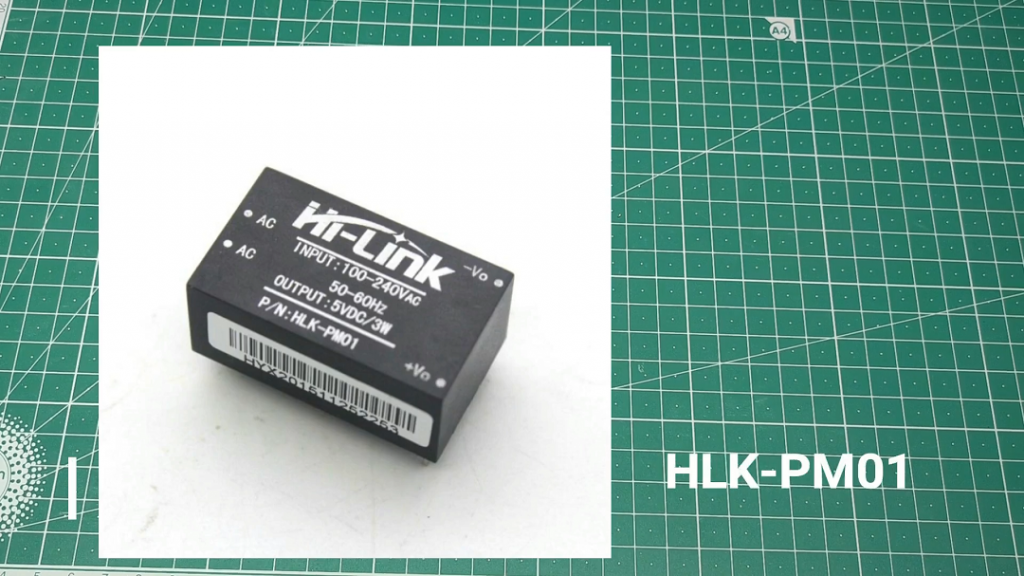
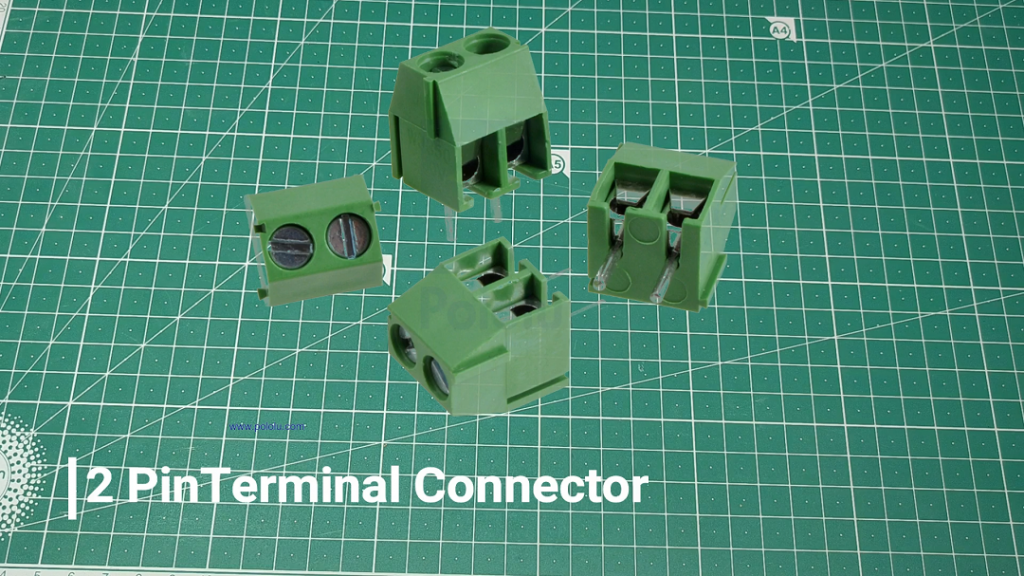
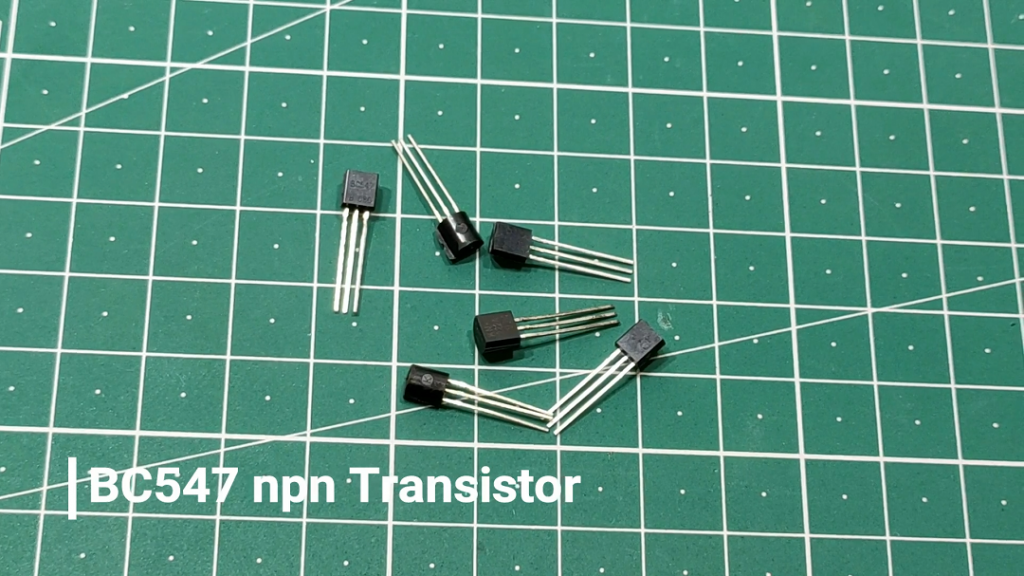
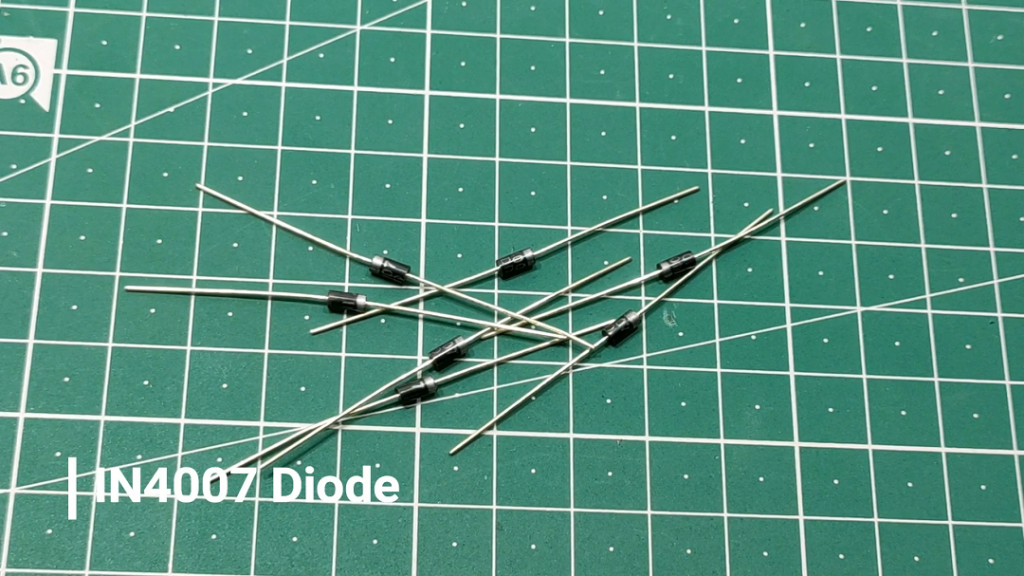
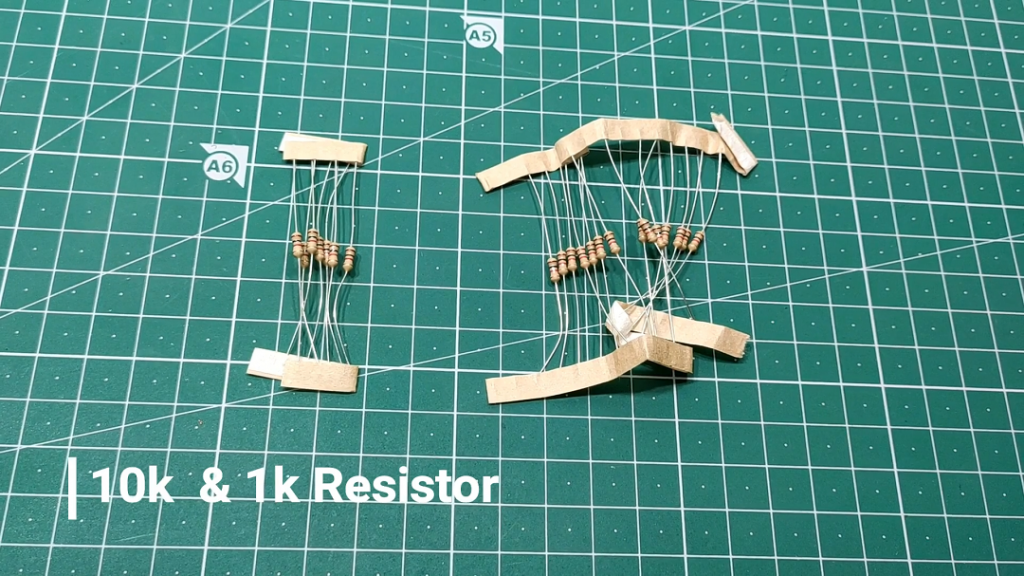
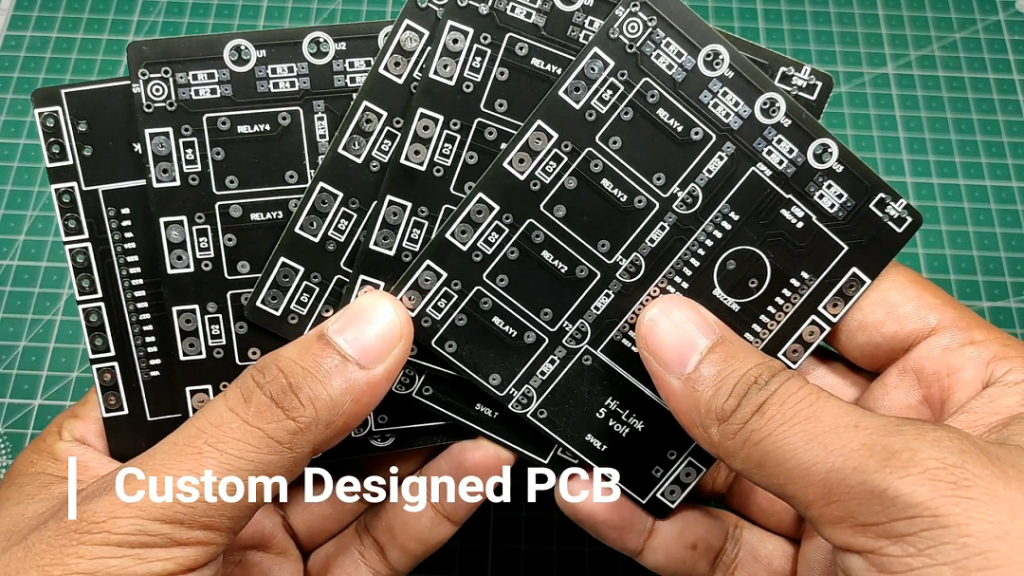
Connection of Switches & Bulbs
Connect switches and bulb as given in diagram below.
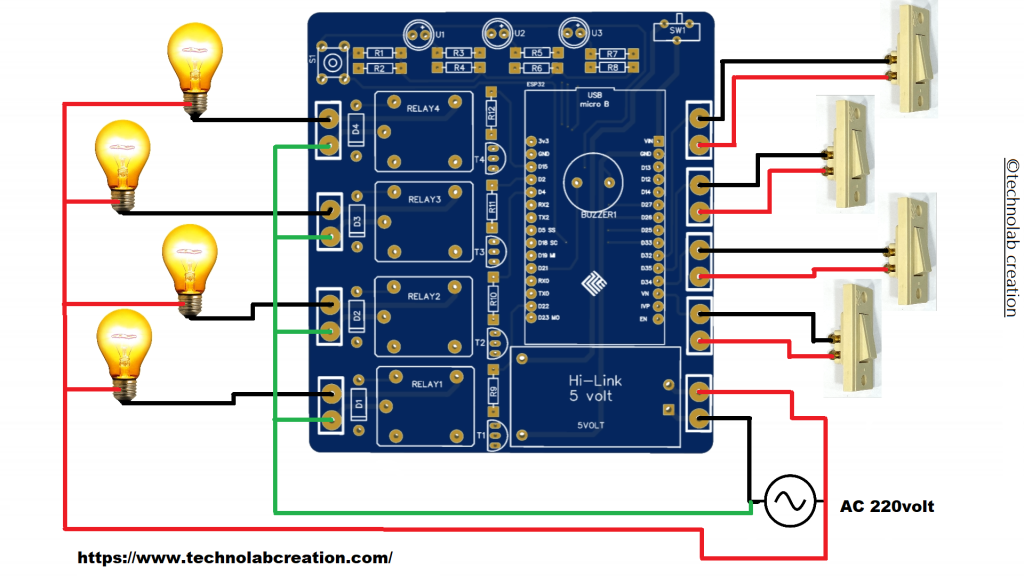
CODE
#include <WiFi.h> // Uncomment this if you are using ESP32 //Web Server App Control Home Automation //#include <ESP8266WiFi.h> //Uncomment this if you are using NodeMCU WiFiClient client; WiFiServer server(80); /* WIFI settings */ const char* ssid = "ENTER YOUR SSID"; //WIFI SSID const char* password = "ENTER YOUR PASSWARD"; //WIFI PASSWORD /* data received from application */ String data =""; #define switch1 32 #define switch2 35 #define switch3 34 #define switch4 39 int Relay1 = 15; int Relay2 = 2; int Relay3 = 4; int Relay4 = 22; int switch_ON_Flag1_previous_I = 0; int switch_ON_Flag2_previous_I = 0; int switch_ON_Flag3_previous_I = 0; int switch_ON_Flag4_previous_I = 0; void setup() { /* initialize motor control pins as output */ pinMode(Relay1, OUTPUT); pinMode(Relay2, OUTPUT); pinMode(Relay3, OUTPUT); pinMode(Relay4, OUTPUT); pinMode(switch1, INPUT); pinMode(switch2, INPUT); pinMode(switch3, INPUT); pinMode(switch4, INPUT); digitalWrite(Relay1,LOW); digitalWrite(Relay2,LOW); digitalWrite(Relay3,LOW); digitalWrite(Relay4,LOW); /* start server communication */ Serial.begin(115200); connectWiFi(); server.begin(); } void loop() { ////////////////////////////////////////////////////////////////// if (digitalRead(switch1) == LOW) { if (switch_ON_Flag1_previous_I == 0 ) { digitalWrite(Relay1, LOW); Serial.println("Relay1- ON"); switch_ON_Flag1_previous_I = 1; } } if (digitalRead(switch1) == HIGH ) { if (switch_ON_Flag1_previous_I == 1) { digitalWrite(Relay1, HIGH); Serial.println("Relay1 OFF"); switch_ON_Flag1_previous_I = 0; } } if (digitalRead(switch2) == LOW) { if (switch_ON_Flag2_previous_I == 0 ) { digitalWrite(Relay2, LOW); Serial.println("Relay1- ON"); switch_ON_Flag2_previous_I = 1; } } if (digitalRead(switch2) == HIGH ) { if (switch_ON_Flag2_previous_I == 1) { digitalWrite(Relay2, HIGH); Serial.println("Relay1 OFF"); switch_ON_Flag2_previous_I = 0; } } if (digitalRead(switch3) == LOW) { if (switch_ON_Flag3_previous_I == 0 ) { digitalWrite(Relay3, LOW); Serial.println("Relay1- ON"); switch_ON_Flag3_previous_I = 1; } } if (digitalRead(switch3) == HIGH ) { if (switch_ON_Flag3_previous_I == 1) { digitalWrite(Relay3, HIGH); Serial.println("Relay1 OFF"); switch_ON_Flag3_previous_I = 0; } } if (digitalRead(switch4) == LOW) { if (switch_ON_Flag4_previous_I == 0 ) { digitalWrite(Relay4, LOW); Serial.println("Relay1- ON"); switch_ON_Flag4_previous_I = 1; } } if (digitalRead(switch4) == HIGH ) { if (switch_ON_Flag4_previous_I == 1) { digitalWrite(Relay4, HIGH); Serial.println("Relay1 OFF"); switch_ON_Flag4_previous_I = 0; } } /* If the server available, run the "checkClient" function */ client = server.available(); if (!client) return; data = checkClient (); Serial.println(data); /************************ Run function according to incoming data from application *************************/ if (data == "Relay1on") { digitalWrite(Relay1,LOW); } else if (data == "Relay1off") { digitalWrite(Relay1,HIGH); } else if (data == "Relay2on") { digitalWrite(Relay2,LOW); } else if (data == "Relay2off") { digitalWrite(Relay2,HIGH); } else if (data == "Relay3on") { digitalWrite(Relay3,LOW); } else if (data == "Relay3off") { digitalWrite(Relay3,HIGH); } else if (data == "Relay4on") { digitalWrite(Relay4,LOW); } else if (data == "Relay4off") { digitalWrite(Relay4,HIGH); } } void connectWiFi() { Serial.println("Connecting to WIFI"); WiFi.begin(ssid, password); while ((!(WiFi.status() == WL_CONNECTED))) { delay(300); Serial.print(".."); } Serial.println(""); Serial.println("WiFi connected"); Serial.println("ESP32 Local IP is : "); Serial.println((WiFi.localIP())); } /********************************** RECEIVE DATA FROM the APP ******************************************/ String checkClient (void) { while(!client.available()) delay(1); String request = client.readStringUntil('\r'); request.remove(0, 5); request.remove(request.length()-9,9); return request; }
Network Credentials
Insert your network credentials in the following variables.
const char* ssid = "ENTER YOUR SSID";
const char* password = "ENTER_YOUR_PASSWORD";
Video Tutorial
Post Views: 16
Where is the mobile Application man